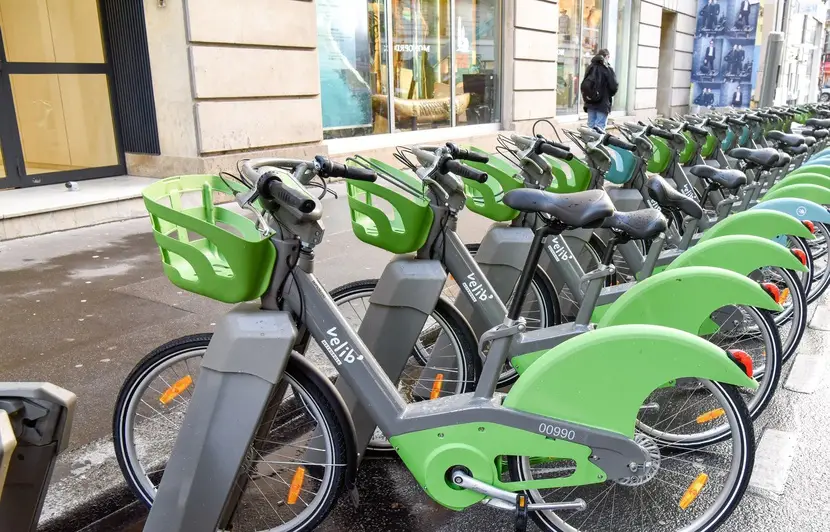
Preparing for a Data Scientist Role
2024, Nov 15
In my preparation for this exciting opportunity as a Data Scientist , I am focusing on mastering the following skills. This article documents my approach to each skill, detailing technical implementations and practical applications.
1. Pandas for Data Processing
Key Tasks:
- Handling Missing Data:
- Replace missing values with the mean/median:
df['column_name'].fillna(df['column_name'].mean(), inplace=True)
- Drop rows with missing data:
df.dropna(subset=['column_name'], inplace=True)
- Forward-fill missing data:
df.fillna(method='ffill', inplace=True)
- Replace missing values with the mean/median:
- Data Transformation:
- Normalize or scale columns:
from sklearn.preprocessing import MinMaxScaler scaler = MinMaxScaler() df['scaled_column'] = scaler.fit_transform(df[['column_name']])
- Normalize or scale columns:
- Removing Duplicates:
df.drop_duplicates(inplace=True)
- Group and Aggregate:
grouped = df.groupby('category_column')['numeric_column'].sum()
2. Manage Data Transfer Between Different Platforms Using Data Transfer Packages (e.g., FTP)
Key Tools:
- Using Python’s
ftplib
for FTP Transfers:- Upload a File:
from ftplib import FTP ftp = FTP('ftp.server.com') ftp.login(user='username', passwd='password') with open('file_to_upload.csv', 'rb') as f: ftp.storbinary('STOR file_to_upload.csv', f) ftp.quit()
- Download a File:
ftp = FTP('ftp.server.com') ftp.login(user='username', passwd='password') with open('downloaded_file.csv', 'wb') as f: ftp.retrbinary('RETR remote_file.csv', f.write) ftp.quit()
- Upload a File:
- Data Transfer with SFTP (Using
pysftp
):import pysftp with pysftp.Connection('hostname', username='user', password='pass') as sftp: sftp.put('local_file.csv', 'remote_file.csv') sftp.get('remote_file.csv', 'local_file.csv')
3. Data Integrity
Ensuring Data Integrity:
- Verify Data Completeness:
assert df.isnull().sum().sum() == 0, "Data contains missing values!"
- Check Duplicates:
assert df.duplicated().sum() == 0, "Data contains duplicates!"
- Validate Data Types:
assert df['date_column'].dtype == 'datetime64[ns]', "Date column is not in the correct format!"
- Use Hashing for Validation:
import hashlib def hash_file(file_path): with open(file_path, 'rb') as f: return hashlib.sha256(f.read()).hexdigest() assert hash_file('file.csv') == 'expected_hash_value', "File integrity compromised!"
4. Relational Data Warehouses
Working with SQL:
- Creating a Task:
CREATE TASK daily_update SCHEDULE 'USING CRON 0 0 * * * UTC' AS SELECT * FROM source_table INTO target_table;
- Creating Views:
CREATE VIEW sales_summary AS SELECT category, SUM(revenue) AS total_revenue FROM sales_data GROUP BY category;
- Writing Complex Queries:
SELECT customer_id, AVG(purchase_amount) AS avg_purchase FROM purchases WHERE purchase_date >= '2023-01-01' GROUP BY customer_id HAVING avg_purchase > 100;
5. Machine Learning (Regression, Classification, Clustering)
Regression (Linear Regression):
- Implementation:
from sklearn.linear_model import LinearRegression model = LinearRegression() model.fit(X_train, y_train) predictions = model.predict(X_test)
- Metrics:
from sklearn.metrics import mean_squared_error print(mean_squared_error(y_test, predictions))
Classification (Logistic Regression):
- Implementation:
from sklearn.linear_model import LogisticRegression clf = LogisticRegression() clf.fit(X_train, y_train) predictions = clf.predict(X_test)
- Metrics:
from sklearn.metrics import classification_report print(classification_report(y_test, predictions))
Clustering (K-Means):
- Implementation:
from sklearn.cluster import KMeans kmeans = KMeans(n_clusters=3) kmeans.fit(data) print(kmeans.labels_)
- Visualization:
import matplotlib.pyplot as plt plt.scatter(data[:, 0], data[:, 1], c=kmeans.labels_) plt.show()
Conclusion
By deeply understanding and practicing these topics, I am equipping myself with the technical knowledge and practical skills required for this role. This preparation ensures I can confidently handle the challenges and responsibilities outlined in the job description.