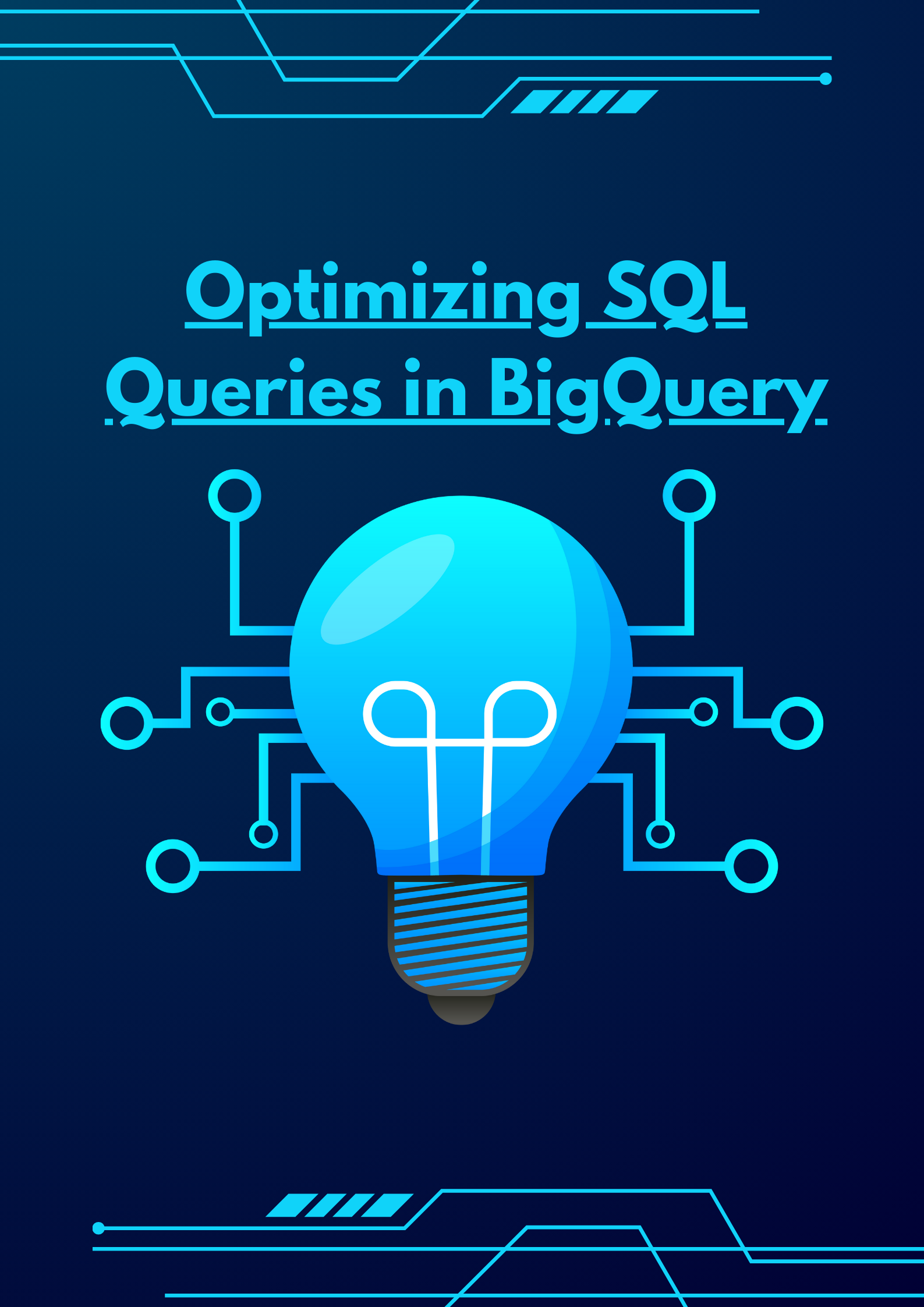
Optimizing SQL Queries in BigQuery
Optimizing SQL Queries in BigQuery: A Deep Dive into Partitioned Tables, CTEs, and Views
In today’s data-driven world, optimizing SQL queries is crucial to ensure performance, reduce costs, and make data handling more efficient. In this article, we will explore advanced techniques for optimizing SQL queries in BigQuery: Partitioned Tables, Common Table Expressions (CTEs), and Views. We’ll break down when and where to use each of these, as well as the benefits they offer.
1. Partitioned Tables in BigQuery
What is a Partitioned Table?
A partitioned table is one that is divided into segments, called partitions, based on specific column values (often a timestamp or date). This allows BigQuery to scan only relevant data during queries, significantly reducing the amount of data processed.
When to Use Partitioned Tables
- Time-based Data: If your dataset contains time-series data (e.g., logs, events), partitioning by a date column is essential. It allows you to focus only on a specific range of dates.
- Large Datasets: When dealing with large datasets, partitioning prevents BigQuery from scanning the entire table unnecessarily.
Best Practices for Partitioning
- Date Partitioning: Partition tables by the
DATE
orTIMESTAMP
column for time-series data. - Integer Partitioning: Partition on integer columns in special cases (e.g., user ID).
- Query Optimization: Use
PARTITION BY
in yourCREATE TABLE
statement:CREATE OR REPLACE TABLE `your_project.dataset.table` PARTITION BY DATE(column_name) AS SELECT * FROM `your_project.dataset.source_table`;
Gains from Using Partitioned Tables
- Cost Savings: BigQuery charges based on the amount of data scanned. Partitioning reduces the scan size, lowering costs.
- Improved Query Performance: Queries that filter based on partitioned columns will only scan relevant partitions, resulting in faster query execution.
2. Common Table Expressions (CTEs)
What is a CTE?
A Common Table Expression (CTE) is a temporary result set that can be referenced within a SELECT
, INSERT
, UPDATE
, or DELETE
statement. CTEs help break down complex queries and improve readability.
When to Use CTEs
- Breaking Down Complex Queries: Use CTEs to split complex queries into smaller, readable chunks.
- Reusing Query Logic: If you need to use the same logic multiple times within a query, CTEs allow you to define the logic once and reuse it.
Best Practices for CTEs
- Limit CTE Usage in Iterative Queries: Since CTEs are recalculated each time they are referenced, use them with caution in complex, iterative queries.
- Chain CTEs: You can chain multiple CTEs to create a clean and organized query structure:
WITH temp_table AS ( SELECT column1, column2 FROM `your_project.dataset.source_table` ), filtered_table AS ( SELECT * FROM temp_table WHERE column2 > 100 ) SELECT * FROM filtered_table;
Gains from Using CTEs
- Improved Query Readability: CTEs make your queries more understandable by breaking down complex logic.
- Code Reusability: You can define transformations once and reuse them multiple times in a query, leading to cleaner code.
3. Views in BigQuery
What is a View?
A view in BigQuery is a saved SQL query that can be referenced as if it were a table. Views can abstract complex queries and create reusable data representations.
When to Use Views
- Abstracting Complexity: Use views to hide complex SQL logic from end users, allowing them to focus on simpler queries.
- Reusable Logic: Views enable you to encapsulate business logic that can be reused across multiple queries or applications.
- Securing Sensitive Data: You can use views to expose only a subset of data to certain users without giving them access to the entire table.
Best Practices for Views
- Use Materialized Views for Frequent Queries: If the underlying data changes infrequently and the query is run often, consider materialized views for faster performance.
- Limit Nested Views: Avoid deeply nested views, as they can negatively impact query performance.
Gains from Using Views
- Query Simplification: Views simplify data access by abstracting complex logic.
- Improved Data Security: Views allow you to expose only the data that users need, improving data security.
4. Combining Partitioned Tables, CTEs, and Views for Maximum Optimization
By combining partitioned tables, CTEs, and views, you can build highly efficient, maintainable, and secure SQL workflows in BigQuery.
Example: Combining Partitioned Tables with CTEs and Views
WITH filtered_data AS (
SELECT column1, column2
FROM `your_project.dataset.partitioned_table`
WHERE _PARTITIONDATE BETWEEN '2024-01-01' AND '2024-01-31'
)
SELECT * FROM filtered_data;
In this example, partitioning reduces the data scanned, the CTE organizes the logic, and a view could be used to reuse this logic elsewhere.
Conclusion
By leveraging partitioned tables, CTEs, and views, you can optimize both the performance and maintainability of your SQL queries in BigQuery. These techniques not only improve query execution times but also provide cost savings and enhanced data security. Apply these best practices to ensure your BigQuery queries are both efficient and scalable.