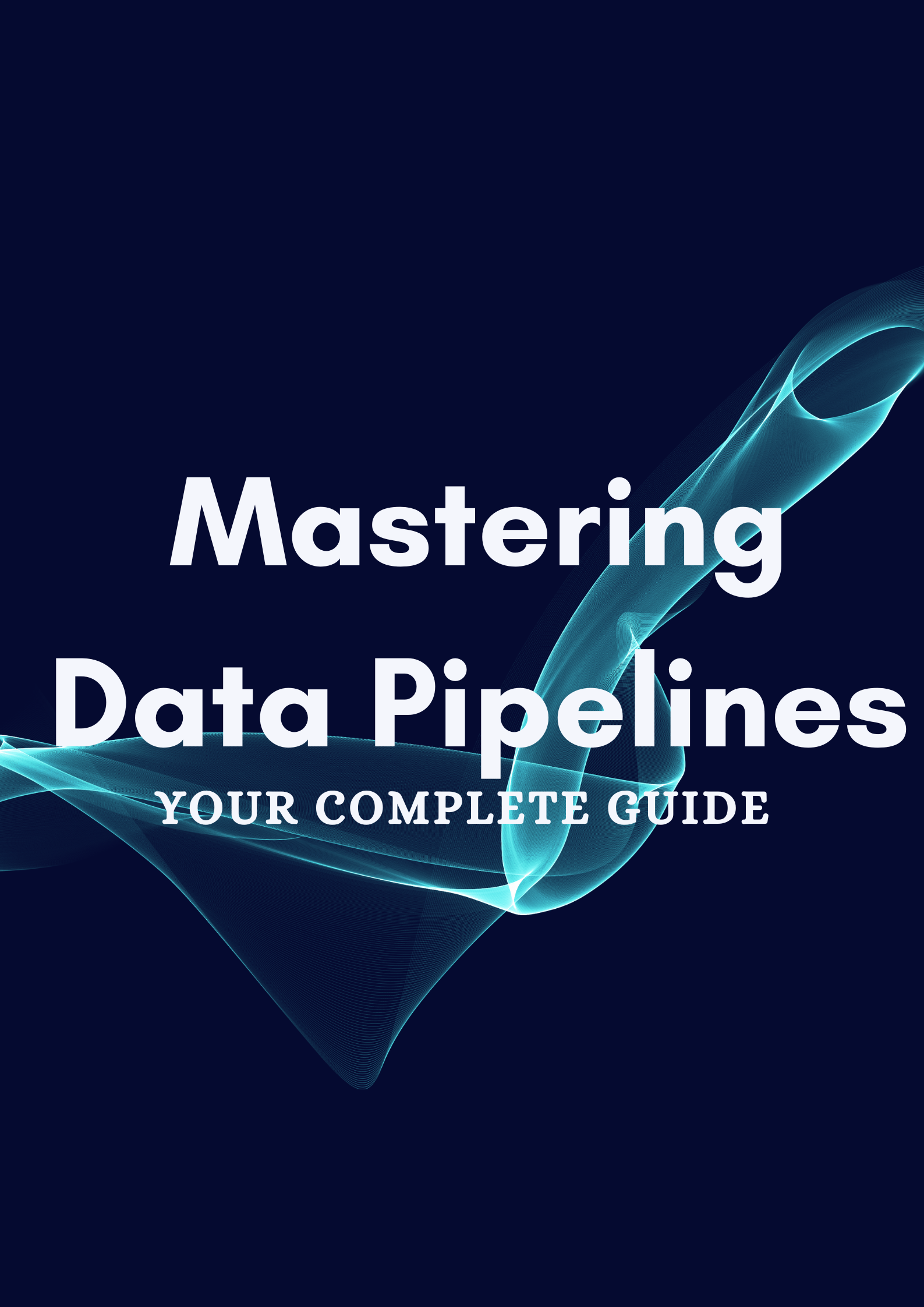
Mastering Data Pipelines
Mastering Data Pipelines: A Step-by-Step Guide Using BigQuery, Dataflow, and Airflow
Data pipelines are essential for processing large volumes of data efficiently. This guide will help you build optimized data pipelines using Google Cloud tools: BigQuery, Dataflow, and Airflow. We’ll cover each step in detail, providing practical advice, security tips, and optimization tricks.
1. Understand the Requirements
Before building your pipeline:
- Data Sources: Identify where your data comes from (e.g., GCS, relational databases, APIs).
- Data Destinations: Know where your data will end up (e.g., BigQuery).
- Transformations: Define the necessary transformations (e.g., filtering, aggregations).
2. Set Up Google Cloud Platform (GCP) Services
You’ll use:
- BigQuery: A data warehouse for storage and analysis.
- Dataflow: A managed service for stream and batch processing.
- Airflow: A workflow orchestration tool (via Cloud Composer).
BigQuery
Create a dataset and table in BigQuery:
CREATE OR REPLACE TABLE `your_project.your_dataset.your_table`
PARTITION BY DATE(_PARTITIONTIME) AS
SELECT * FROM `source_table`
WHERE date BETWEEN '2024-01-01' AND '2024-01-31';
Optimization Tip: Use partitioned tables to reduce query costs and improve performance. Clustered columns can further optimize frequent filtering.
Dataflow
Configure a Dataflow pipeline for transformation and streaming:
import apache_beam as beam
from apache_beam.options.pipeline_options import PipelineOptions
def run():
pipeline_options = PipelineOptions()
p = beam.Pipeline(options=pipeline_options)
(p
| 'ReadData' >> beam.io.ReadFromText('gs://your-bucket/input.csv')
| 'TransformData' >> beam.Map(lambda row: transform_fn(row))
| 'WriteToBigQuery' >> beam.io.WriteToBigQuery(
'your_project:your_dataset.your_table',
schema='column1:STRING, column2:INTEGER',
write_disposition=beam.io.BigQueryDisposition.WRITE_TRUNCATE
)
)
p.run()
Optimization Tip: Use autoscaling for dynamic resource allocation and manage costs by adjusting worker count based on traffic volume.
Airflow (Cloud Composer)
Orchestrate your pipeline with Airflow:
from airflow import DAG
from airflow.utils.dates import days_ago
from airflow.providers.google.cloud.operators.dataflow import DataflowStartFlexTemplateOperator
with DAG('data_pipeline', start_date=days_ago(1), schedule_interval='@daily') as dag:
start_dataflow_job = DataflowStartFlexTemplateOperator(
task_id="start_dataflow_job",
template_path="gs://your-bucket/dataflow_template.json",
location='us-central1',
parameters={
'input': 'gs://your-bucket/input.csv',
'output': 'your_project:your_dataset.your_table'
}
)
Optimization Tip: Break complex pipelines into multiple DAGs and use Sensors to manage dependencies and ensure smooth operations.
3. Data Transformation and Optimization
Use Apache Beam transformations (e.g., Map
, FlatMap
, GroupByKey
) in Dataflow for efficient data processing.
Optimization Tip: Avoid shuffle operations like GroupByKey
where possible, as they can be resource-intensive.
Security Best Practice: Encrypt data at rest and in transit. Ensure that your data is transmitted via HTTPS and encrypted using GCP’s default encryption.
4. Monitoring and Error Handling
Best Practice: Use Stackdriver (now Cloud Monitoring) for logging and monitoring the pipeline. Set up alerts for pipeline latency or errors.
Security Tip: Monitor API usage and restrict access to logs to ensure only authorized personnel can view sensitive data.
5. Cost Optimization
- Use partitioned tables in BigQuery to avoid scanning unnecessary data.
- Enable Dataflow FlexRS (Flexible Resource Scheduling) for cost-efficient batch processing.
6. Security Best Practices
- IAM Roles and Service Accounts: Grant only the necessary permissions to users.
- Encryption: Ensure data encryption both in transit and at rest.
- Data Masking: Mask sensitive data before loading it into BigQuery.
7. Best Practices for Maintenance
- Version Control: Keep your pipeline definitions in Git to track changes and maintain rollback options.
- Documentation: Document your DAGs and transformations for clarity, especially for complex processes.
- Automation: Use Airflow’s scheduling features to automate data refreshes efficiently.
By following these steps, you will build a highly optimized, secure, and scalable data pipeline using BigQuery, Dataflow, and Airflow. Performance optimization and security are key to ensuring your pipeline runs smoothly and remains compliant with data regulations.